Actors
|
|
Xakep | Дата: Вторник, 28 Мая 2019, 17:16 | Сообщение # 21 |
めちゃくちゃちゃ
Сейчас нет на сайте
| Понятно, согласен в общем-то. Вообще интересный проект, ECS я люблю, время от времени задумываюсь над тем, чтобы вернуться в геймдев, если все таки надумаю попробую этот фреймворк, звездочку на гитхабе поставил, так что не потеряется
|
|
| |
pixeye | Дата: Суббота, 15 Июня 2019, 14:54 | Сообщение # 22 |
Red Winter Software
Сейчас нет на сайте
| у гитхаба в бете запущена программа спонсоров. В общем суть, что любой желающий сможет поблагодарить опенсорщиков парой долларов : ) типа патреона ток на гитхабе. Сейчас собирают претедентов на бетку. Буду признателен если проголосуете за меня : ) мой профиль если что.
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
|
|
| |
pixeye | Дата: Пятница, 28 Июня 2019, 11:52 | Сообщение # 23 |
Red Winter Software
Сейчас нет на сайте
| Cryoshock Mini для тех кто интересуется темой ECS - обновляю учебный проект на фреймворке c учетом изменений.
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
Сообщение отредактировал pixeye - Пятница, 28 Июня 2019, 11:58 |
|
| |
pixeye | Дата: Четверг, 04 Июля 2019, 20:45 | Сообщение # 24 |
Red Winter Software
Сейчас нет на сайте
| Добавил поддержку распараллеливания для рассчетов : )
Код namespace Pixeye.Source { sealed class ProcessorTest : Processor, ITick { public Group<ComponentPosition> groupThreaded;
public ProcessorTest() { groupThreaded.MakeConcurrent(100000, Environment.ProcessorCount - 1, HandleCalculation); }
public void Tick(float delta) { if (Input.GetKeyDown(KeyCode.Alpha1)) { for (int i = 0; i < 500000; i++) { var e = Entity.Create(); e.Set<ComponentPosition>(); } }
// One thread if (Input.GetKeyDown(KeyCode.Alpha2)) { for (int i = 0; i < groupThreaded.length; i++) { ref var entity = ref groupThreaded.entities[i]; ref var cPosition = ref entity.ComponentPosition(); for (int j = 0; j < 1000; j++) { cPosition.pos.x += 1 * delta; } } }
// MultiThread if (Input.GetKeyDown(KeyCode.Alpha3))
groupThreaded.Execute(delta); }
static void HandleCalculation(SegmentGroup segment) { for (int i = segment.indexFrom; i < segment.indexTo; i++) { ref var entity = ref segment.source.entities[i]; ref var cPosition = ref entity.ComponentPosition(); for (int j = 0; j < 1000; j++) { cPosition.pos.x += 1 * segment.delta; } } } } }
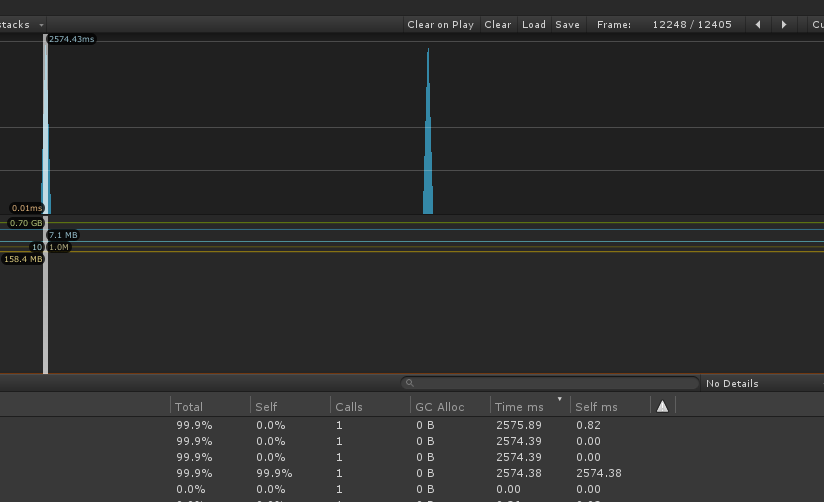
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
Сообщение отредактировал pixeye - Четверг, 04 Июля 2019, 20:46 |
|
| |
pixeye | Дата: Суббота, 31 Августа 2019, 00:34 | Сообщение # 25 |
Red Winter Software
Сейчас нет на сайте
| Выкатил новую версию (2019.08.30) фреймворка.
- Добавлены процессоры с автогруппами - Переработаны и оптимизированы события групп сущностей - Переработан и оптимизирован настроечный код для компонентов.
Больше об изменениях почитать можно тут.
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
Сообщение отредактировал pixeye - Суббота, 31 Августа 2019, 10:07 |
|
| |
pixeye | Дата: Понедельник, 16 Сентября 2019, 11:38 | Сообщение # 26 |
Red Winter Software
Сейчас нет на сайте
| Версия 2019.9.15
Потребует рефакторинг компонентов. Был существенно упрощен boilerplate код для них.
Код static partial class component { // нужно для exclude фильтра групп. Опционально. public const string health = "Pixeye.Source.ComponentHealth";
public static ref ComponentHealth ComponentHealth(in this ent entity) => ref StorageComponentHealth.components[entity.id]; }
sealed class StorageComponentHealth : Storage<ComponentHealth> { // создает новый компонент. Быстрее чем new T(); public override ComponentHealth Create() => new ComponentHealth(); // Когда компоненты на кадре умирают их можно почистить через этот метод. Опционально. public override void Dispose() { for (int i = 0; i < disposedLen; i++) { ref var component = ref components[disposed[i]]; component.val = 0; component.valMax = 0; } } }
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
Сообщение отредактировал pixeye - Понедельник, 16 Сентября 2019, 11:45 |
|
| |
pixeye | Дата: Понедельник, 30 Сентября 2019, 16:47 | Сообщение # 27 |
Red Winter Software
Сейчас нет на сайте
| 
Как работаю с 2д анимациями на Unity
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
Сообщение отредактировал pixeye - Понедельник, 30 Сентября 2019, 16:48 |
|
| |
pixeye | Дата: Понедельник, 02 Декабря 2019, 11:46 | Сообщение # 28 |
Red Winter Software
Сейчас нет на сайте
| 
Пишем свой обработчик твинов
ACTORS - мой фреймворк на Unity Until We Die - игра над которой работаю
|
|
| |